Top 5 Best insights from Bjarne Stroustrup on Embedded C++ programming
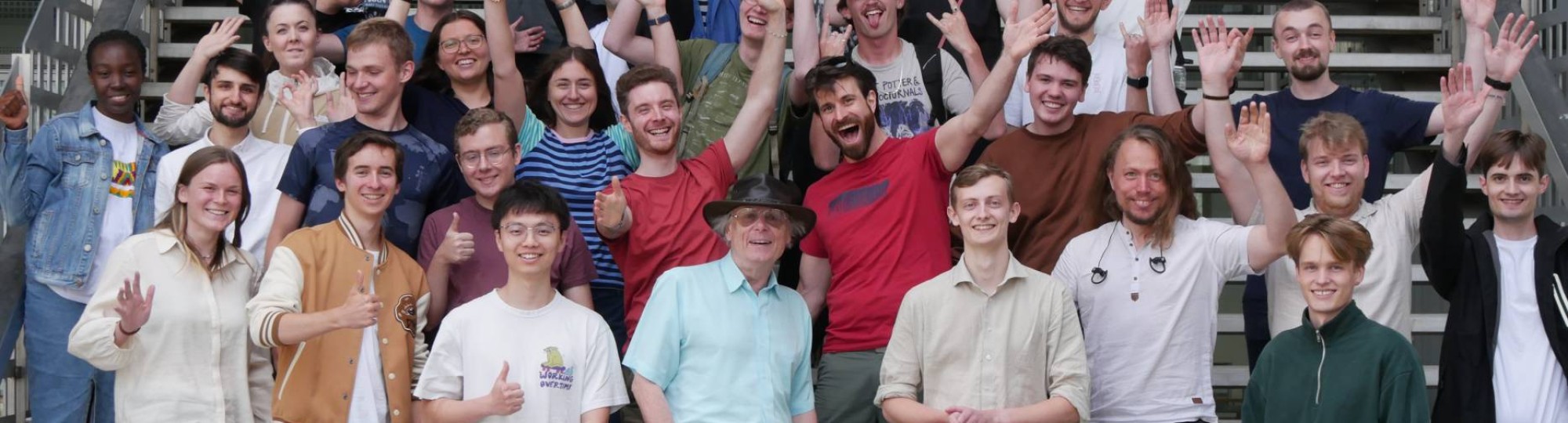
Last summer, Richard Kroesen, HAN Embedded Systems Engineering student, had an unforgettable experience every C++ enthusiast dreams of: being taught by Bjarne Stroustrup. He is the creator of the C++ programming language. Richard is happy to share the insights he learned with you!
Richard, along with other Embedded Systems Engineering students, got the chance to explore the latest and greatest features of contemporary C++ (version 26) during a two-week summer school in Aarhus Denmark. He also had the opportunity to learn directly from the person who invented the language. ‘This only happens to you once in a lifetime, right?’
In addition, this programme offered him the opportunity to gain international knowledge in a short, intensive course. ‘Perfect for those of us who cannot take a full semester abroad,’ he says.
Richard is happy to share the most exciting insights he gained from this course. ‘These are my personal insights, so you may encounter these topics in a different way as you dive deeper into C++, specifically within the context of embedded systems. Hopefully this will make you as excited about new concepts in C++ as I am!’
These are my personal insights, so you may encounter these topics in a different way as you dive deeper into C++
1. Generic Programming: Making Code Reusable and Powerful
One of the first things we covered was generic programming, which is essential in creating scalable and reusable code. The idea is to make your code flexible by using templates, allowing you to operate with different data types without having to rewrite the same logic repeatedly.
For example, if you are designing a dashboard for an embedded device. Let’s say a simple layout with buttons and a background. You can create templates for the shapes and sizes of the UI elements. At compile-time, you can specify the properties (like size, color, and position), which you would not modify at runtime.
Here is a small example of a generic function in C++:
template <typename T>
return a + b;
}
With this, you can add integers, floats, or even custom data types. All in one go! Bjarne emphasized that templates are one of the keys to writing efficient, reusable code, and it was eye-opening to see how this concept can simplify complex systems. The purpose of this principle is to building your programs in an enhanced flexible and scalable way. On Embedded targets the benefit is that at-compile-time based program is more deterministic as well.
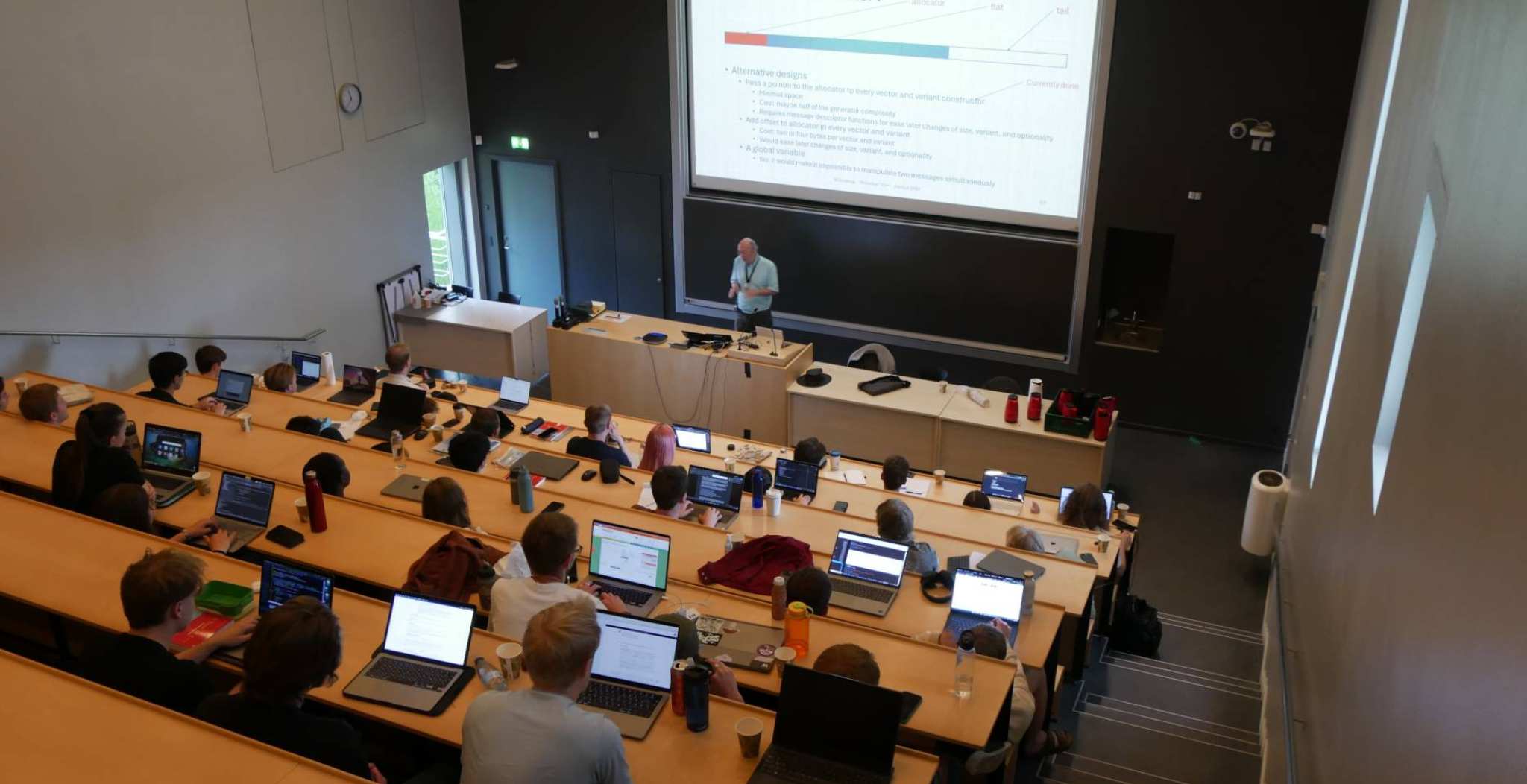
2. RAII: Mastering Resource Management
Next up, we tackled the powerful principle of RAII (Resource Acquisition Is Initialization). It’s all about controlling resources like memory, data access objects for external connectivity and related objects. The purpose of this approach is to properly clean the objects that are not needed any longer.
Bjarne showed us how this concept plays a critical role in avoiding memory leaks and crashes. Especially in embedded systems this could cause a catastrophic device failure. With profound consequences, think of failure in critical aviation systems.
With the use of RAII, C++ ensures that allocated resources are automatically released when an object goes out of scope. It is brilliant, right?
Practical take aways:
- Use Smart Pointers, never have a raw pointer (aka naked pointer)
- Use the newest semantics and mechanisms of the cpp language
Keep in mind that the drawback is that there is additional memory overhead therefore, still this is not that much on top. Bjarne explained that this simple, yet powerful technique is one of the reasons C++ remains highly efficient for resource-constrained applications. You are still making use of raw pointers performance but with additional protection mechanism.
3. Writing Maintainable Code: Bjarne’s Philosophy
Bjarne has a very clear philosophy on how C++ should be written: the code should be both readable and efficient. He stressed the importance of making your code not just work, but also easy to maintain and extend. This means writing in small, modular components, which helps you avoid “spaghetti code” and makes future updates much easier.
By breaking your code into smaller, self-contained pieces, you make it easier to debug and test each part. Write your functions which make sense in the context, and do not need any comments.
4. Lightweight Error Handling in Embedded Systems
One of the challenges in embedded systems is handling errors efficiently and practically. Unlike desktop applications, you cannot afford the luxury of bulky logging systems or throwing exceptions all over the place. Instead, Bjarne suggested using lightweight error-handling methods, such as returning error codes or using optional types.
An example with std::optional:
std::optional
if (b == 0) {
return std::nullopt; // Do not return a value.
}
return a / b;
Bjarne also recommends creating a hierarchical error handling, such that the errors are pushed to the higher layers which contain the logic about what to do when such error occurs.
This approach keeps the error handling simple and efficient, which is crucial when working on systems with limited processing power and memory, like embedded devices. It was fascinating to hear from Bjarne himself how C++ is evolving to support such use cases.
5. Firmware-optimalization: C++m to the Rescue
Finally, we dove into code performance optimization, an important topic when building large scale. In resource-constrained environments, every bit of performance counts, and Bjarne shared valuable tips on optimizing C++ code for speed and memory efficiency.
One key strategy is to minimize dynamic memory allocation. In other words, avoid using new or malloc unless necessary. Instead, favor stack allocation or pre-allocated buffers to prevent memory fragmentation and reduce runtime overhead.
Bjarne also talked about the power of constexpr and inline functions for at-compile-time optimizations. By letting the compiler evaluate certain expressions ahead of time, you save precious processing cycles during execution.
Quick example of constexpr in action:
constexpr int square(const int x) {
return x * x;
}
In the course we did more optimalizations on search algorithms of vectors, lists, array, and set but this is less relevant for embedded devices. So for this reason I would not go into detail, if you are curious about the difference feel free to checkout my repository on my GitHub page.
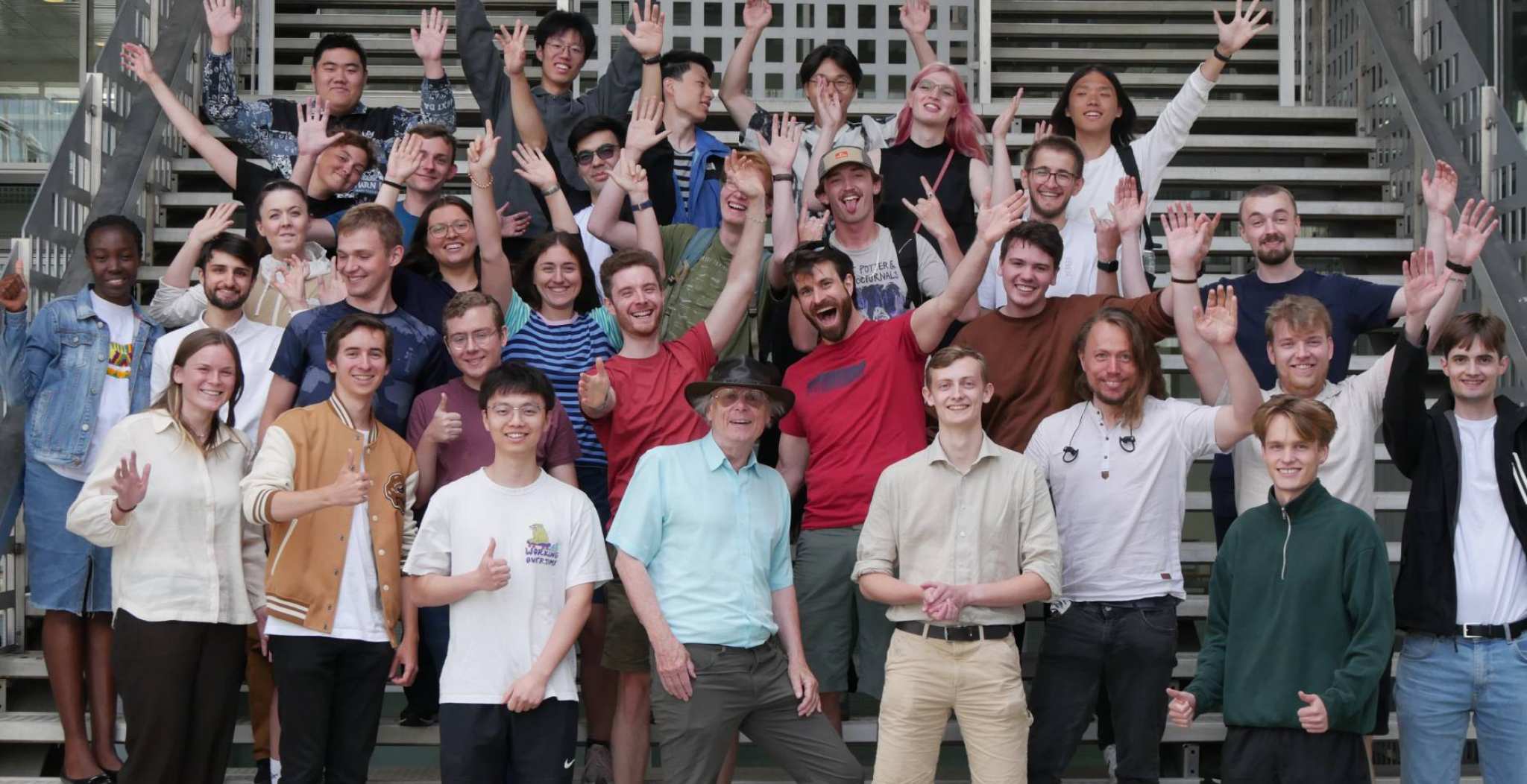
Conclusion: A Once-in-a-Lifetime Learning Experience
Learning C++ directly from Bjarne Stroustrup was an experience I will never forget. The insights I gained on generic programming, RAII, error handling, and optimization have completely changed the way I approach coding in C++. And I hope this blog sparks inspiration for you too to try the newest features and approaches!
If you are just starting your C++ journey, do not be intimidated by these new features! Start with the fundamentals, experiment, and most importantly, keep learning. C++ is an incredibly powerful tool, and mastering its modern features will set you on the path to becoming a proficient, confident programmer.
Learn more?
Are you ready to dive into the world of modern C++? If you have any questions reach to me through my LinkedIn (Richard Kroesen).